Display
This module controls the 5×5 LED display on the front of your board. It can be used to display images, animations and even text.
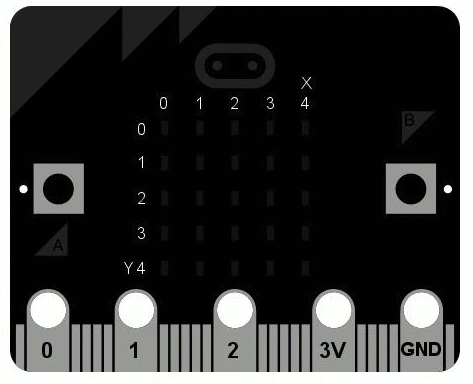
Functions
- microbit.display.get_pixel(x, y)
Return the brightness of the LED at column
x
and rowy
as an integer between 0 (off) and 9 (bright).
- microbit.display.set_pixel(x, y, value)
Set the brightness of the LED at column
x
and rowy
tovalue
, which has to be an integer between 0 and 9.
- microbit.display.clear()
Set the brightness of all LEDs to 0 (off).
- microbit.display.show(image)
Display the
image
.
- microbit.display.show(image, delay=400, \*, wait=True, loop=False, clear=False)
If
image
is a string, float or integer, display letters/digits in sequence. Otherwise, ifimage
is an iterable sequence of images, display these images in sequence. Each letter, digit or image is shown withdelay
milliseconds between them.If
wait
isTrue
, this function will block until the animation is finished, otherwise the animation will happen in the background.If
loop
isTrue
, the animation will repeat forever.If
clear
isTrue
, the display will be cleared after the iterable has finished.Note that the
wait
,loop
andclear
arguments must be specified using their keyword.
Note
If using a generator as the iterable
, then take care not to allocate any memory
in the generator as allocating memory in an interrupt is prohibited and will raise a
MemoryError
.
- microbit.display.scroll(text, delay=150, \*, wait=True, loop=False, monospace=False)
Scrolls
text
horizontally on the display. Iftext
is an integer or float it is first converted to a string usingstr()
. Thedelay
parameter controls how fast the text is scrolling.If
wait
isTrue
, this function will block until the animation is finished, otherwise the animation will happen in the background.If
loop
isTrue
, the animation will repeat forever.If
monospace
isTrue
, the characters will all take up 5 pixel-columns in width, otherwise there will be exactly 1 blank pixel-column between each character as they scroll.Note that the
wait
,loop
andmonospace
arguments must be specified using their keyword.
- microbit.display.on()
Use on() to turn on the display.
- microbit.display.off()
Use off() to turn off the display (thus allowing you to re-use the GPIO pins associated with the display for other purposes).
- microbit.display.is_on()
Returns
True
if the display is on, otherwise returnsFalse
.
- microbit.display.read_light_level()
Use the display’s LEDs in reverse-bias mode to sense the amount of light falling on the display. Returns an integer between 0 and 255 representing the light level, with larger meaning more light.
Example
To continuously scroll a string across the display, and do it in the background, you can use:
import microbit
microbit.display.scroll('Hello!', wait=False, loop=True)